JavaScript 101, for Programmers
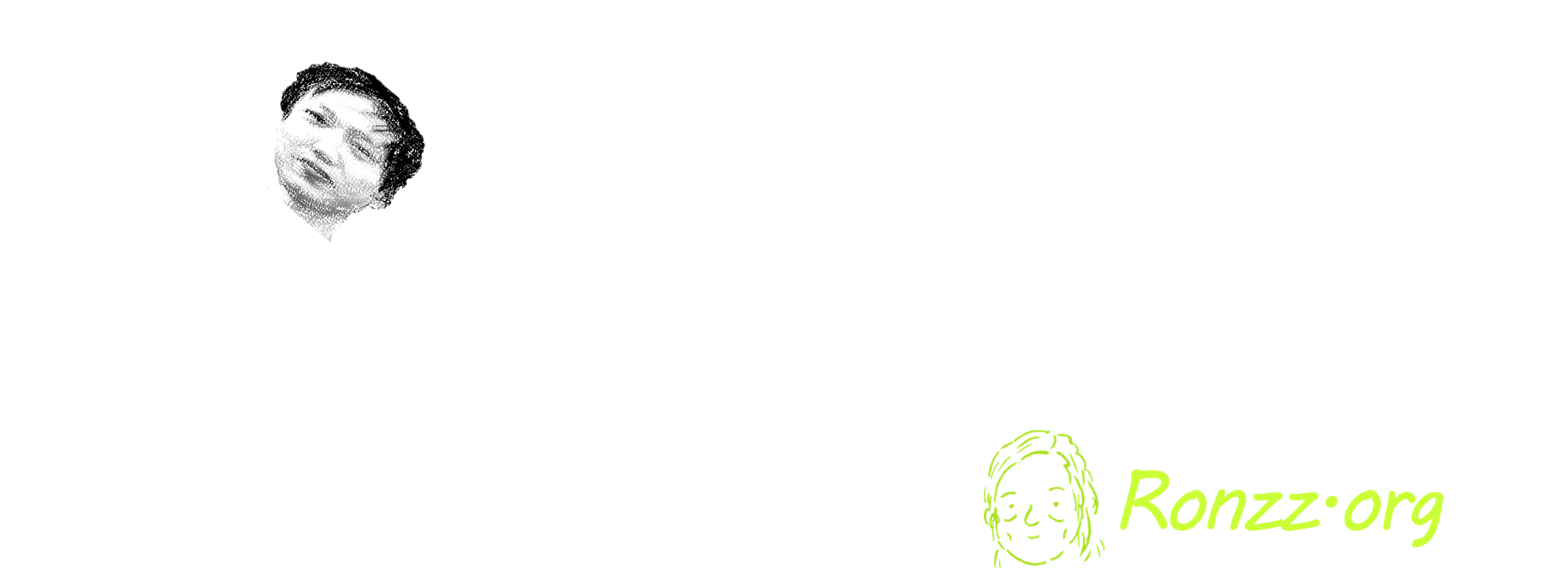
This article is part of my web development series 'webDEV101'. You can read the whole series here:
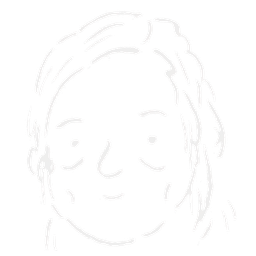
JavaScript?
JavaScript is THE scripting language of the internet, designed to work together with HTML and CSS to create web animations and web-based applications. Continuing the LEGO analogy we have been using with HTML and CSS, Javascript is like the motors, sensors, and computer elements in Lego Technic sets, bringing life to the otherwise inanimate structures.
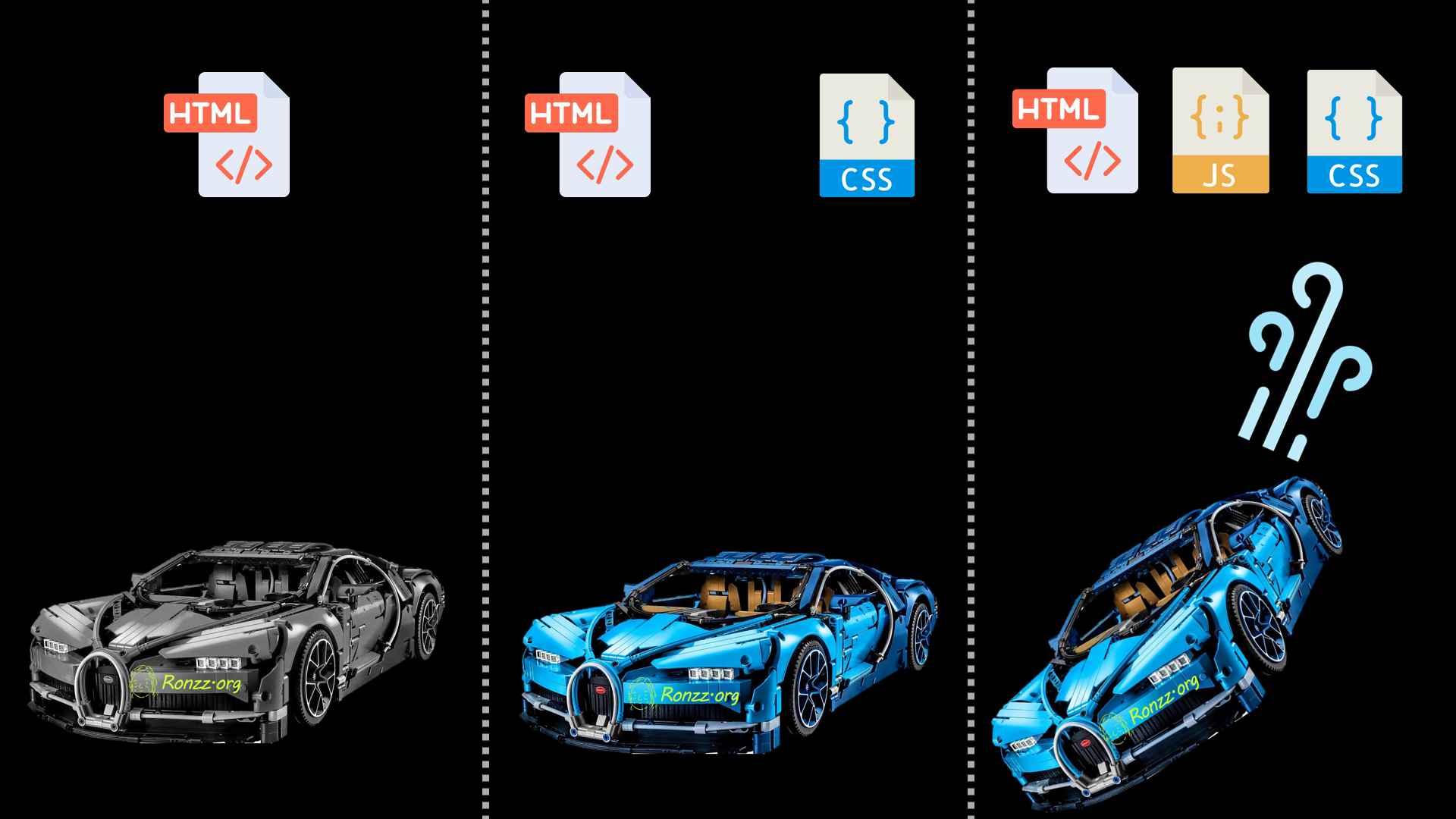
Basic syntax
/*
Sample JavaScript file
*/
var globalVar = "I am a global variable";
function varExample() {
var functionVar = "I am a function variable";
if (true) {
var functionVarVar2 = "I am still a function variable";
}
}
console.log(globalVar);
// console.log(functionVar); > Uncaught ReferenceError: functionVar is not defined
if (true) {
let blockVar = "I am a block variable";
console.log(blockVar);
}
// console.log(blockVar); > Uncaught ReferenceError: blockVar is not defined
// Global constant
const PI = 3.14159;
// PI = 3.14; > Uncaught TypeError: Assignment to constant variable
const obj = { key: "value" };
obj.key = "new value"; // If constant IS object/array > properties/elements modifications possible
console.log(obj.key); // Output: new value
Begin line comments with //
. Enclose block comments between /*
and */
End each statement with ;
Enclose strings with either ' '
or " "
the .
Print to the console via console.log([variable]);
(Don't lose the semicolon!)
Constants & Variables
var globalVar = "I am a global variable";
Declare global variables with var outside any {}
.
function varExample() {
var functionVar = "I am a function variable";
if (true) {
var functionVarVar2 = "I am still a function variable";
}
}
Declare function variables with var anywhere inside the function {}
.
function varExample() {
var functionVar = "I am a function variable";
if (true) {
var functionVarVar2 = "I am still a function variable";
let blockVar = "I am a block variable";
}
}
Declare block variables, which are accessible only within the {}
block where they are defined, with let
.
var hey = 2048;
hey = 'haha';
JavaScript variables are dynamically typed. So, a variable initially assigned an integer value can later be assigned a string value without redeclaration.
Constants
// Global constant
const PI = 3.14159;
// PI = 3.14;> Uncaught TypeError: Assignment to constant variable
const obj = { key: "value" };
obj.key = "new value"; // If constant IS object/array > properties/elements modifications possible
console.log(obj.key); // > new value
Constants must be initialised upon declaration, and cannot be modified once declared.
However, if a constant is an array or object, its elements or properties can be later modified.
Complex data types: Objects and arrays
An object is a collection of unordered keypairs. Each keypair denotes a property of the object:
{ name: 'Sandwich', calories: 250 }
An array is an ordered collection of values. It is possible to construct an array of objects:
const foodItems = [
{ name: 'Apple', calories: 95 },
{ name: 'Banana', calories: 105 },
{ name: 'Orange', calories: 62 },
{ name: 'Sandwich', calories: 250 }
];
``
Or even an array of arrays:
const Items_nested = [clothesItems, foodItems];
An array of arrays is called a multi-dimensional array.
An interesting tool is the spread operator '...':
const Items_nested = [clothesItems, foodItems];
const Items = [...clothesItems, ...foodItems];
While line 1 is constructing a multi-dimensional array, line 2 is concatenating the two arrays and equivalent to:
const Items=[
{ name: 'Apple', calories: 95 },
{ name: 'Banana', calories: 105 },
{ name: 'Orange', calories: 62 },
{ name: 'Sandwich', calories: 250 },
{ name: 'T-shirt', size: 'M', color: 'Blue' },
{ name: 'Jeans', size: '32', color: 'Black' },
{ name: 'Jacket', size: 'L', color: 'Green' },
{ name: 'Hat', size: 'One Size', color: 'Red' }
];
Camel casing
var camelCaseVariables
When naming variables, the JavaScript convention is 'camel case', which means capitalising the first letter of each successive word except the first one for multi-word variables.
Hoisting
hoistedFunction(); // > I am hoisted
function hoistedFunction() {
console.log("I am hoisted");
}
console.log(hoistedVar); // > undefined
var hoistedVar = "I am hoisted";
console.log(hoistedVar); // > I am hoisted
JavaScript variables are hoisted to the top of their scope during compilation. Therefore, calling a variable before its declaration in the source code does not necessarily lead to an error.
However, only the variable declaration, not the variable definition, is hoisted.
What
var hoistedVar = "I am hoisted";
is doing is actually two things:
var hoistedVar
hoistedVar = "I am hoisted";
declaration and definition.
Only the declaration is hoisted, so the value of 'hoistedVar' will be 'undefined' when logged before 'var hoistedVar = "I am hoisted
Avoid reliance on hoisting for readability and predictability.
Float value comparators
Operator | Description | Example |
---|---|---|
== |
Equal to | a == b |
=== |
Strictly equal to | a === b |
!= |
Not equal to | a != b |
!== |
Strictly not equal to | a !== b |
< |
Less than | a < b |
<= |
Less than or equal to | a <= b |
> |
Greater than | a > b |
>= |
Greater than or equal to | a >= b |
For, forEach, while, and do...while loop
for Loop
for (let i = 0; i < 5; i++) {
console.log(i); // > 0, 1, 2, 3, 4
}
for (initial condition, test condition, increment){
//function
}
forEach Loop
// Example array of food items
const foodItems = [
{ name: 'Apple', calories: 95 },
{ name: 'Banana', calories: 105 },
{ name: 'Orange', calories: 62 },
{ name: 'Sandwich', calories: 250 }
];
// Function to calculate total calories
function calculateTotalCalories(items) {
let totalCalories = 0;
items.forEach(item => {
totalCalories += item.calories;
});
return totalCalories;
}
// Using the function
const totalCalories = calculateTotalCalories(foodItems);
console.log(`Total Calories: ${totalCalories}`); // Output: Total Calories: 512
The forEach
loop is a specialised for
loop for iteration over arrays.
It has the syntax 'array'.forEach(array_element => { //function });
while Loop
while (true){
console.log('ronzz.org')
}
do ... while Loop
do {
console.log("Ronzz.org"); // > Ronzz.org
} while (false);
With do ... while
loop the enclosed code is executed once blindly before the condition is tested. Therefore, even though the condition is always false in the example, 'Ronzz.org' will be printed once.
Switch
if (id<=2 ) {
//
}
else if (id==3 ) {
//
}
else {
//
}
Regular expression in JavaScript
// Example of using regex to validate an email address
const email = 'test@example.com';
const emailRegex = /^[^\s@]+@[^\s@]+\.[^\s@]+$/;
console.log(emailRegex.test(email)); // true
// Example of using regex to find all numbers in a string
const stringWithNumbers = 'There are 123 apples and 456 oranges.';
const numberRegex = /\d+/g;
const numbers = stringWithNumbers.match(numberRegex);
console.log(numbers); // ['123', '456']
// Example of using regex to replace all spaces with underscores
const stringWithSpaces = 'Hello World Hello Heaven!';
const spaceRegex = /\s/g;
const stringWithUnderscores = stringWithSpaces.replace(spaceRegex, '_');
console.log(stringWithUnderscores); // 'Hello_World_Hello_Heaven!'
Regular expressions are enclosed between a pair of /
. After the closing /
, one or more optional switches can be added to modify the matching behaviour:
g
: Global search: Match all instances instead of just the first one.i
: Case-insensitive search: Ignore capitalisation differencesm
: Multiline mode:^
and$
anchors match the start and end of each line, respectively, rather than the start and end of the entire string.s
: DotAll mode: Normally, a dot matches everything except newline characters. With 's' newline characters are matched.u
: Unicode modey
: Sticky mode: Activates the 'lastIndex' property. When called each subsequent time, matching starts after the previous match instead of the beginning, hence avoiding repetition.
As seen, regular expressions can be used in JavaScript to verify input, extract information, and systematically replace parts of a string.
Can you think of some practical applications in web development?
Practical application: Web development
HTML is hard-coding:
Bienvenue!
Hey! Do you love this guide?
Consider subscribing! There is a free tier!
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Example HTML Document</title>
</head>
<body>
<header>
<h1 id="header">Bienvenue!</h1>
</header>
<main>
<button id="Chinese">See this page in Chinese</button>
<section>
<h2 id="subheader">Hey! Do you love this guide?</h2>
<p id="paragraph">Consider subscribing! There is a free tier!</p>
</section>
</main>
<footer>
<p>© 2025 Ronzz.org</p>
<img src="https://ronzz.org/content/images/2025/02/tp_Ronzz.org_logo.png">
</footer>
<script src="Language_switch.js"></script>
</body>
</html>
All the text in <title>,<h1,h2>
, and <p>
etc., are static and cannot be changed later with HTML.
So, what if we need to change those texts later? For instance, if we are building a multi-lingual webpage, and the user has clicked on the language switch, do we need to redirect them to another URL, potentially reloading all the resources ? If the webpage has a considerable amount of graphics and audio, that will be painfully inefficient!
That is when JavaScript comes in.
JavaScript can access HTML elements by id
:
const header = document.getElementById('header');
Note: this method returns only the 1st match. It is as such essential that all the 'id's in your HTML is unique.
And change the text in the accessed element:
header.textContent = '欢迎!'
Or do both in one step:
document.getElementById('header').textContent = '欢迎!';
According to pre-defined trigger conditions:
document.getElementById('Chinese').addEventListener('click', function() {
document.getElementById('header').textContent = '欢迎!';
document.getElementById('subheader').textContent = '嘿!你喜欢这个指南吗?';
document.getElementById('paragraph').textContent = '考虑订阅吧!有一个免费层!';
});
The EventListener method listens for user input. Whenever the user clicks the button identified by id= Chinese
, the header text changes.
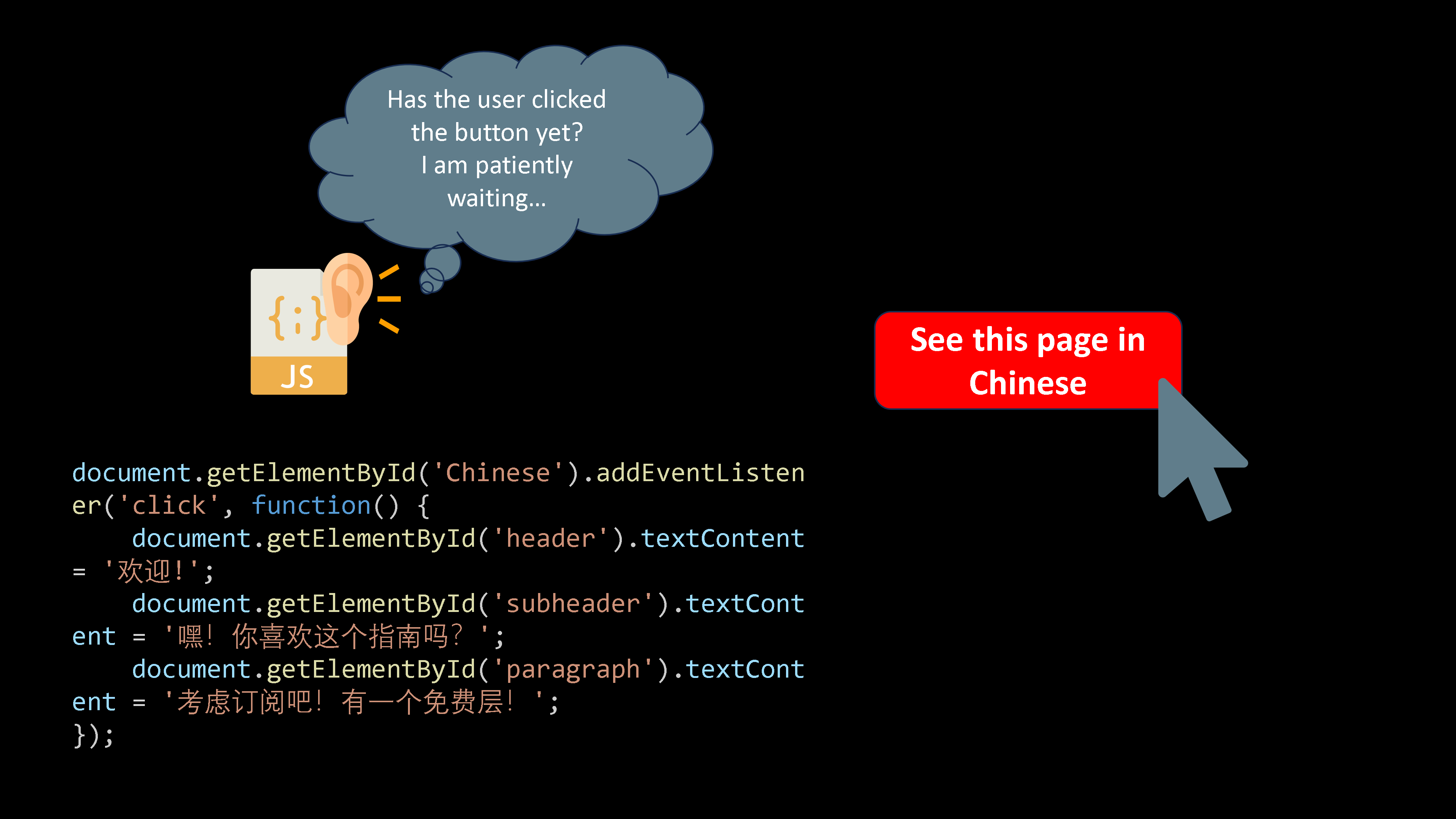
Bienvenue!
Hey! Do you love this guide?
Consider subscribing! There is a free tier!
Exercise:
Can you enhance the switch to allow users to switch back to English after switching to Chinese, in case it was done by accident?)
Practical application: Web application
Leveraging HTML and JavaScript, one can build various web applications, ranging from email clients to online document/photo editors to social media.
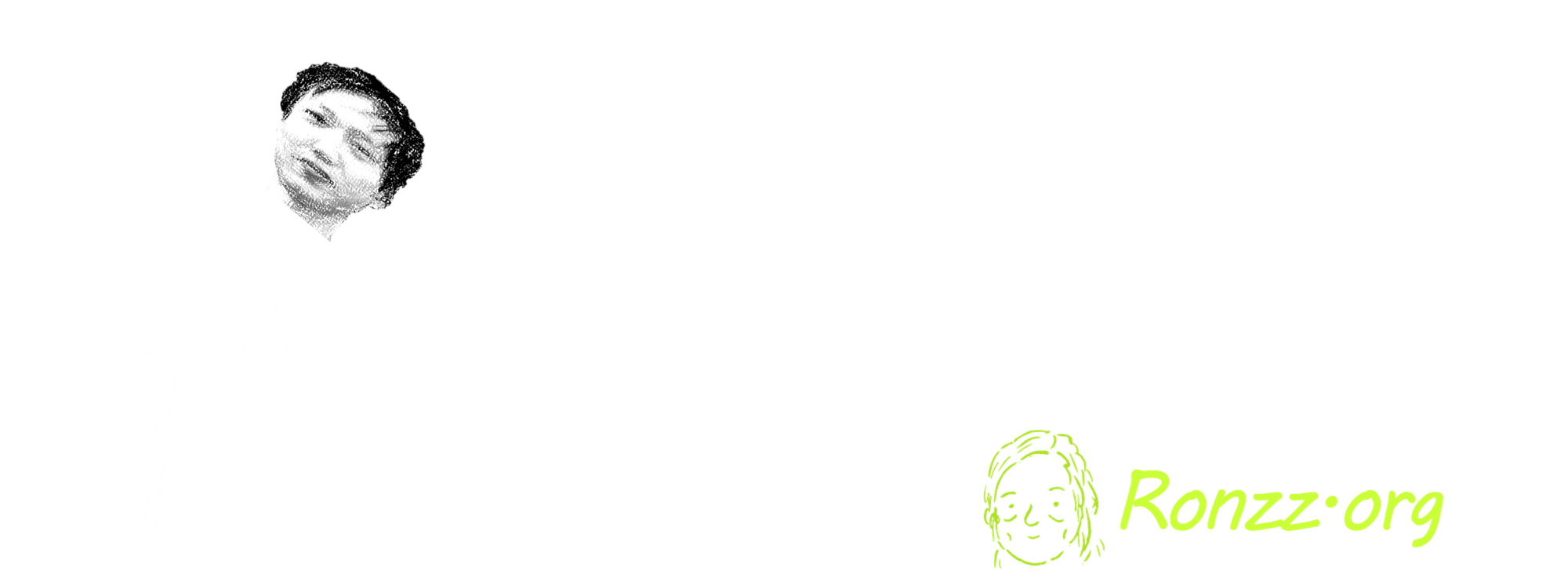
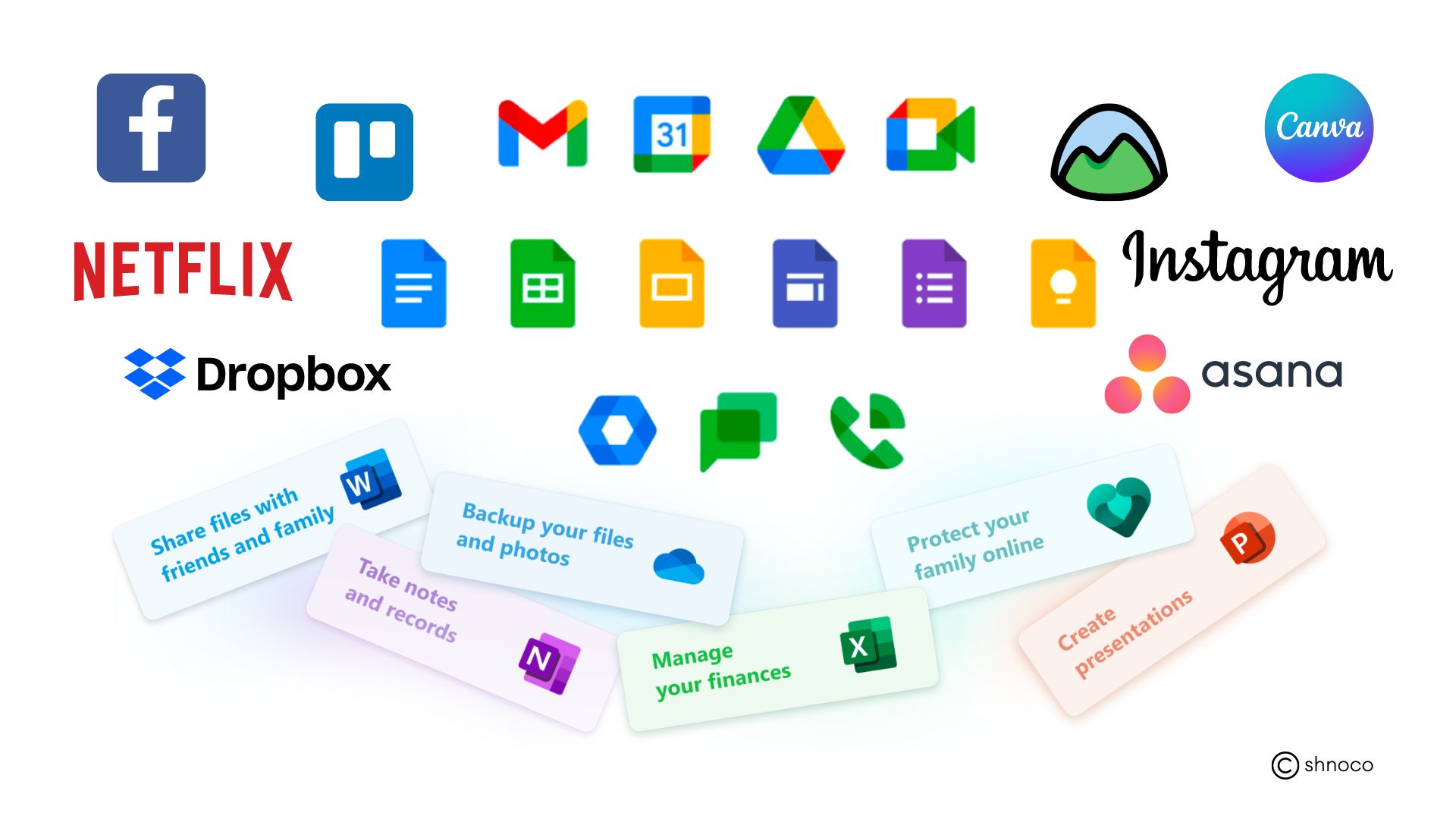
Below is a simple web application: a cashier calculator to aggregate item prices, apply promotions, calculate the final price, and export the detailed data in a .csv
file, which can be parsed into a receipt generator.
Cashier Calculator
Notice the Javascript is embedded in the HTML file via <script></script>
, which can be convenient for short scripts.
Most methods used in the script are already explained in previous sections of this article. Much of the rest is self-explanatory. We will focus on the few less intuitive bits:
Line 45-60:
const itemContainer = document.createElement('div');
itemContainer.classList.add('item-container');
itemContainer.innerHTML = `
<fieldset>
<legend>Item</legend>
<label for="item">Item name:</label>
<input type="text" class="item-name" name="item">
<label for="price">Price:</label>
<input type="number" min="0" class="item-price" name="price">
<label for="quantity">Quantity:</label>
<input type="number" min="0" class="item-quantity" name="quantity">
<label for="discount">Discount:</label>
<input type="number" min="0" max="100" class="item-discount" name="discount">
<label for="discount">%</label>
</fieldset>
`;
These lines create an HTML element and store it as the constant itemContainer
. The .classlist.add
method in 2 adds attribute class='item_container'
to the newly created <div>
. The .innerHTML
method in 3 specifies the HTML content in the <div>
.
These lines on their own do nothing. itemContainer
must be wrapped in an event listener to be triggered by specific conditions and dynamically inject content into the HTML file accordingly. See line 44,61,62:
document.getElementById('add-item').addEventListener('click', function() {
...
document.getElementById('all-item-containers').appendChild(itemContainer);
});
The .appendChild
method injects itemContainer
as a child element of the parent element id=add-item
.
Line 86:
let csvContent = "data:text/csv;charset=utf-8,";
This line creates a blank CSV data string, with utf-8
encoding. All files, images, videos, spreadsheets, etc., can be stored as data strings, either directly or as encoded binaries.
Line 92:
const price = parseFloat(container.querySelector('.item-price').value) || 0;
This line parses the value
of the item-price
input field as a float. ||
is the OR operator. If the parse result is invalid, then price
is set as 0
.
Line 102-108:
const encodedUri = encodeURI(csvContent);
const link = document.createElement('a');
link.setAttribute('href', encodedUri);
link.setAttribute('download', 'cashier_data.csv');
document.body.appendChild(link);
link.click();
document.body.removeChild(link);
encodeURI
escapes all the special characters in the CSV data string to ensure it can be parsed in a URL. Then createElement
in 2 create an HTML anchor element. setAttribute
in 3-4 link the anchor element to the encoded CSV data string, and specify to download content found at the URL and name the file cashier_data.csv
once the anchor is activated. 5-7 temporarily add the anchor to the <body>
of the HTML for it to be automatically clicked, triggering the download.
Exercise:
Notice the Reset
button clears everything. Can you add a delete item button to delete just one particular item?
Remarks
If you followed this tutorial, you should now be familiar with Javascript syntax and basic methods. To learn more, consider finding a few interesting, simple JS projects on GitHub, and explore the source code. GitHub Copilot can answer most of your questions concerning unfamiliar methods.
Copyright Statement
Énoncé du droit d'auteur
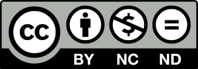
Much of our content is freely available under the Creative Commons BY-NC-ND 4.0 licence, which allows free distribution and republishing of our content for non-commercial purposes, as long as Ronzz.org is appropriately credited and the content is not being modified materially to express a different meaning than it is originally intended for. It must be noted that some images on Ronzz.org are the intellectual property of third parties. Our permission to use those images may not cover your reproduction. This does not affect your statutory rights.
Nous mettons la plupart de nos contenus disponibles gratuitement sous la licence Creative Commons By-NC-ND 4.0, qui permet une distribution et une republication gratuites de notre contenu à des fins non commerciales, tant que Ronzz.org est correctement crédité et que le contenu n'est pas modifié matériellement pour exprimer un sens différent que prévu à l'origine.Il faut noter que certaines images sur Ronzz.org sont des propriétés intellectuelles de tiers. Notre autorisation d'utiliser ces images peut ne pas couvrir votre reproduction. Cela n'affecte pas vos droits statutaires.Statement
Member discussion